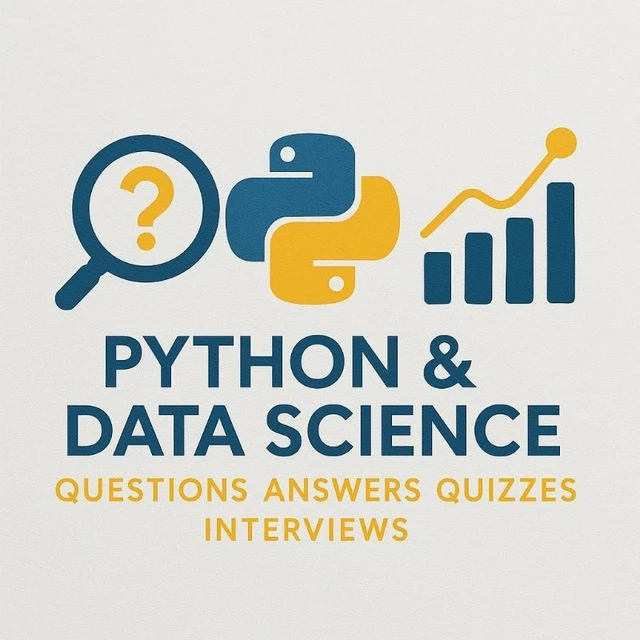
DA
Python Data Science Jobs & Interviews
https://t.me/datascienceq
–
subscribers
0.67%
ER (week)
1.17%
ERR (week)
Description
Your go-to hub for Python and Data Science—featuring questions, answers, quizzes, and interview tips to sharpen your skills and boost your career in the data-driven world.