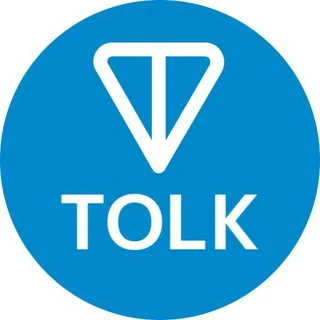
TO
TOLK lang
https://t.me/tolk_lang
–
подписчиков
-
ER за неделю
-
ERR за неделю
Описание
Channel devoted to TOLK — "next-generation FunC" — a language for writing smart contracts in TON